HelloGraphs示例#
示例展示了如何制作简单的2D条形图和线形图。
BarGraph#
示例中的第一个图是条形图。创建它首先是使用GraphsView组件并设置一个适用于暗背景的主题。此主题调整图表背景网格、轴线和标签。
要将其改为条形图,添加一个BarSeries.
系列的X轴是一个具有3个类别的BarCategoryAxis
。我们隐藏了垂直网格和轴线。系列的Y轴是ValueAxis
,其可视范围在20到100之间。将tickInterval
属性设置为每10个值显示主要刻度,并将minorTickCount
属性设置为9,这意味着在每个主要刻度之间将会有9个次要刻度。
然后使用BarSet.
将数据添加到BarSeries
中。这里有3个柱状图,我们定义了自定义的柱状颜色和边框属性。这些属性将覆盖AbstractSeries
可能设置的任何主题。
LineGraph#
示例中的第二个图表是折线图。它也是从定义一个GraphsView
元素开始的。创建了一个自定义的GraphTheme
以获得自定义外观。GraphTheme
为背景网格和坐标轴提供了相当广泛的定制可能性,这些设置在colorTheme
之后应用。
使用自定义的Marker
组件来可视化数据点。
之前的柱状图没有定义单独的SeriesTheme
,因此它使用默认主题。这个折线图使用了一个自定义主题,具有所需的线条颜色。
为了使其成为折线图,添加一个LineSeries.
第一个系列为此图表定义了axisX
和axisY
。它还设置了pointMarker
以使用之前创建的自定义Marker
组件。数据点使用XYPoint
元素添加。
第二个折线系列与第一个类似。由于图表已经包含了它们,因此无需定义axisX
和axisY
。作为GraphsView
中的第二个LineSeries
,自动选择了seriesTheme
中的第二个颜色。
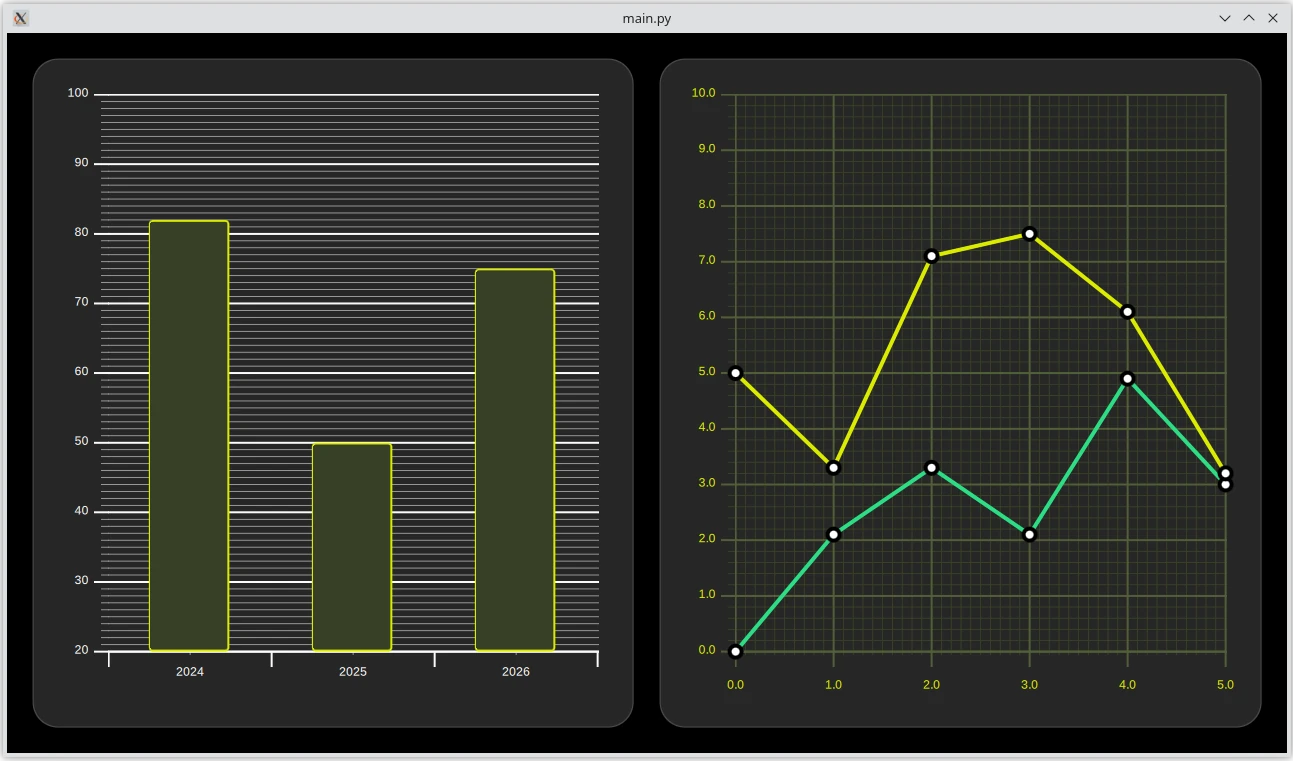
# Copyright (C) 2024 The Qt Company Ltd.
# SPDX-License-Identifier: LicenseRef-Qt-Commercial OR BSD-3-Clause
"""PySide6 port of the Qt Hello Graphs example from Qt v6.x"""
from pathlib import Path
import sys
from PySide6.QtGui import QGuiApplication
from PySide6.QtQuick import QQuickView
if __name__ == '__main__':
app = QGuiApplication(sys.argv)
viewer = QQuickView()
viewer.engine().addImportPath(Path(__file__).parent)
viewer.setColor("black")
viewer.loadFromModule("HelloGraphs", "Main")
viewer.show()
r = app.exec()
del viewer
sys.exit(r)
// Copyright (C) 2024 The Qt Company Ltd.
// SPDX-License-Identifier: LicenseRef-Qt-Commercial OR BSD-3-Clause
import QtQuick
import QtQuick.Layouts
import QtGraphs
Item {
id: mainView
width: 1280
height: 720
RowLayout {
id: graphsRow
readonly property real margin: mainView.width * 0.02
anchors.fill: parent
anchors.margins: margin
spacing: margin
Rectangle {
Layout.fillHeight: true
Layout.fillWidth: true
color: "#262626"
border.color: "#4d4d4d"
border.width: 1
radius: graphsRow.margin
//! [bargraph]
GraphsView {
anchors.fill: parent
anchors.margins: 16
theme: GraphTheme {
colorTheme: GraphTheme.ColorThemeDark
}
//! [bargraph]
//! [barseries]
BarSeries {
axisX: BarCategoryAxis {
categories: [2024, 2025, 2026]
gridVisible: false
minorGridVisible: false
}
axisY: ValueAxis {
min: 20
max: 100
tickInterval: 10
minorTickCount: 9
}
//! [barseries]
//! [barset]
BarSet {
values: [82, 50, 75]
borderWidth: 2
color: "#373F26"
borderColor: "#DBEB00"
}
//! [barset]
}
}
}
Rectangle {
Layout.fillHeight: true
Layout.fillWidth: true
color: "#262626"
border.color: "#4d4d4d"
border.width: 1
radius: graphsRow.margin
//! [linegraph]
GraphsView {
anchors.fill: parent
anchors.margins: 16
theme: GraphTheme {
readonly property color c1: "#DBEB00"
readonly property color c2: "#373F26"
readonly property color c3: Qt.lighter(c2, 1.5)
colorTheme: GraphTheme.ColorThemeDark
gridMajorBarsColor: c3
gridMinorBarsColor: c2
axisXMajorColor: c3
axisYMajorColor: c3
axisXMinorColor: c2
axisYMinorColor: c2
axisXLabelsColor: c1
axisYLabelsColor: c1
}
//! [linegraph]
//! [linemarker]
component Marker : Rectangle {
width: 16
height: 16
color: "#ffffff"
radius: width * 0.5
border.width: 4
border.color: "#000000"
}
//! [linemarker]
//! [lineseriestheme]
SeriesTheme {
id: seriesTheme
colors: ["#2CDE85", "#DBEB00"]
}
//! [lineseriestheme]
//! [lineseries1]
LineSeries {
id: lineSeries1
theme: seriesTheme
axisX: ValueAxis {
max: 5
tickInterval: 1
minorTickCount: 9
labelDecimals: 1
}
axisY: ValueAxis {
max: 10
tickInterval: 1
minorTickCount: 4
labelDecimals: 1
}
width: 4
pointMarker: Marker { }
XYPoint { x: 0; y: 0 }
XYPoint { x: 1; y: 2.1 }
XYPoint { x: 2; y: 3.3 }
XYPoint { x: 3; y: 2.1 }
XYPoint { x: 4; y: 4.9 }
XYPoint { x: 5; y: 3.0 }
}
//! [lineseries1]
//! [lineseries2]
LineSeries {
id: lineSeries2
theme: seriesTheme
width: 4
pointMarker: Marker { }
XYPoint { x: 0; y: 5.0 }
XYPoint { x: 1; y: 3.3 }
XYPoint { x: 2; y: 7.1 }
XYPoint { x: 3; y: 7.5 }
XYPoint { x: 4; y: 6.1 }
XYPoint { x: 5; y: 3.2 }
}
//! [lineseries2]
}
}
}
}
module HelloGraphs
Main 1.0 Main.qml